LeetCode: turning a failed interview into an opportunity for success
- Raf Y
- Dec 31, 2023
- 5 min read
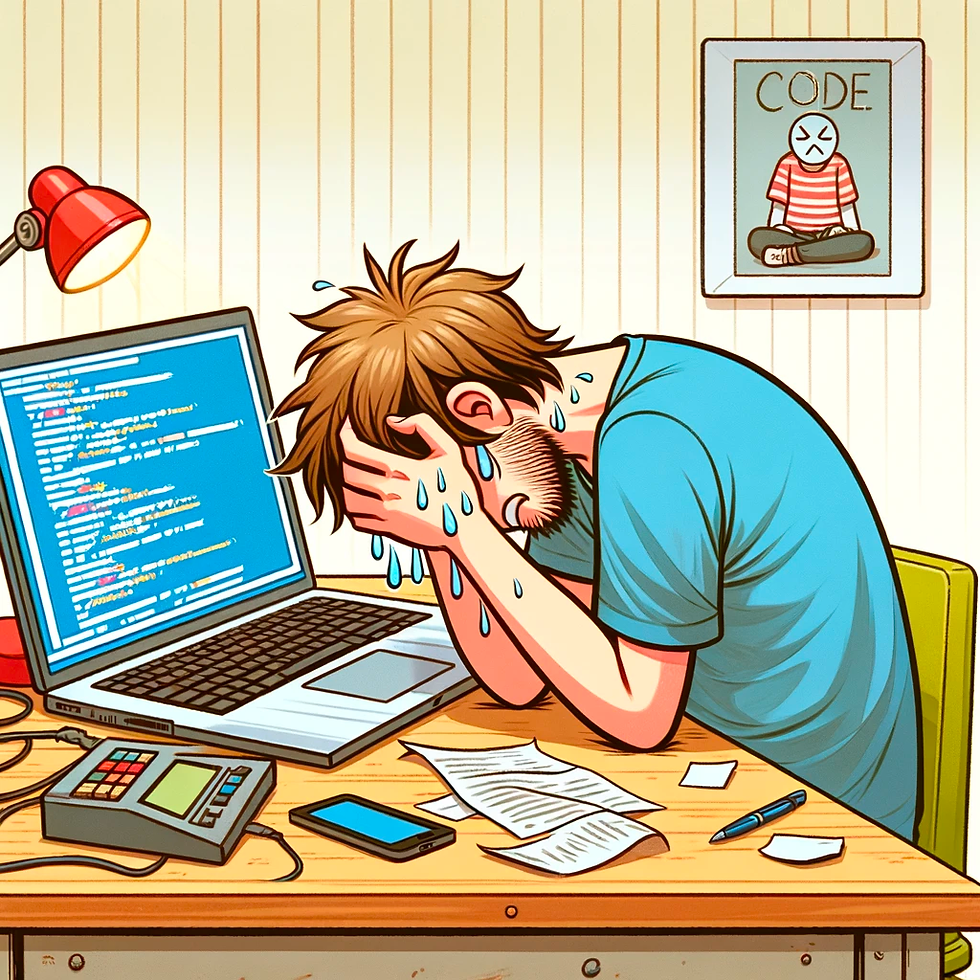
Shortly after having been laid off, I was lucky enough to land a few interviews (reminder that some people out there are going months without landing any serious interviews). In one of those interviews, I advanced to the technical portion - the live coding challenge. Needless to say (and the reason why this post exists!) the technical portion did not go as planned and I was told later that day that I failed the technical interview and that I will not be moving forward in the interview process.
While initially disheartening, this moment turned out to be a pivotal wake-up call. As a Senior Engineer at my last company, I felt very technically apt and capable. This was reinforced by my managers, co-workers, and even others whom were senior to me such as tech leads and principal engineers of the company. However, on a day to day basis, as a Software Developer, you're generally not tied up in the Big-O space and time complexity of the algorithm that you're coding. You rely on the libraries that you use and trust the author to use the right data structures and algorithms to ensure maximum efficiency (this deserves its own blog post and something engineers should not always assume).
The coding challenge made me realize that there’s always room for improvement, and sometimes, we need such experiences to push us and better ourselves. This blog post marks the beginning of journey into the world of LeetCode and interview prep. It's one where I embrace continuous learning and growth.
It will serve a dual purpose: it will be my personal repository, tracking my progress and learnings, and a resource for others on a similar path. If you're preparing for job interviews or looking to improve your coding skills, I hope my journey brings you insights and encouragement. You are not alone in this!
We all face setbacks, but it's how we respond to them that matters. Let's learn and grow together, transforming challenges into opportunities for success.
Right now, my strategy to solve as many "easy" LeetCode problem as I can and log them here. I'll do my best to write up explanations for some of my answers - however there are dedicated YouTube channels and websites that will do a far better job than me! Also, my answers are going to be focused on readability (most of the time) and not efficiency. I am a bit believer of readability & maintainability over convoluted code that might be slightly more efficient (that being said, if there is a marked improvement in efficiency, then that is always better for the business and should be used in a production environment).
I am currently working through the list of "Top Interview 150". I am excited to share my results here and work through them with you as I work through them!
Now - to get into the first problem from Top Interview 150. Question 88 asks:
You are given two integer arrays nums1 and nums2, sorted in non-decreasing order, and two integers m and n, representing the number of elements in nums1 and nums2 respectively.
Merge nums1 and nums2 into a single array sorted in non-decreasing order.
The final sorted array should not be returned by the function, but instead be stored inside the array nums1. To accommodate this, nums1 has a length of m + n, where the first m elements denote the elements that should be merged, and the last n elements are set to 0 and should be ignored. nums2 has a length of n.
Example 1:
Input: nums1 = [1,2,3,0,0,0], m = 3, nums2 = [2,5,6], n = 3
Output: [1,2,2,3,5,6]
Explanation: The arrays we are merging are [1,2,3] and [2,5,6].
The result of the merge is [1,2,2,3,5,6] with the underlined elements coming from nums1.
Example 2:
Input: nums1 = [1], m = 1, nums2 = [], n = 0
Output: [1]
Explanation: The arrays we are merging are [1] and [].
The result of the merge is [1].
Example 3:
Input: nums1 = [0], m = 0, nums2 = [1], n = 1
Output: [1]
Explanation: The arrays we are merging are [] and [1].
The result of the merge is [1].
Note that because m = 0, there are no elements in nums1. The 0 is only there to ensure the merge result can fit in nums1.
My solution:
/**
Do not return anything, modify nums1 in-place instead.
*/
1 function merge(nums1: number[], m: number, nums2: number[], n: number): void {
2 let index = 0;
3 while (index < n) {
4 nums1[index + m] = nums2[index];
5 index++;
6 }
7 nums1.sort((a, b) => a-b)
8 };
On line 2, I am setting a variable index that starts at 0 - this variable will be used to iterate over the nums1 array.
On line 3, I'm setting up a while loop that will iterate over both the nums1 and nums2 arrays. The condition in the while loop is setup to it will continue iterating while index < n. Remember that n is the length of the nums2 array.
Line 4 is the juiciest part: I'm referencing the element at position index + m in the nums1 array. The reason why I'm starting at this offset is because we are told that m is the length of the array of nums1 - remember that to accommodate the merging of the arrays, "nums1 has a length of m + n" - so that means we are essentially starting at positon m and iterating all the way out to array position n - so we are filing of the n portion of the nums1 array. On the right hand side of line 4, I am referencing nums2[index] - remember that nums2 has a length of n, which is what our while loop is set to iterate to.
The rest of the solution is fairly trivial - line 5 I am incrementing index and line 7 I am sorting the array in increasing order as required by the question.
Conclusion:
I did not find this question easy!
Cons of this solution:
On line 7, we the time complexity is O((m+n) log(m+n)) since we are sorting the array nums1 in increasing order. Actually it's O(n + (m+n) log(m+n)) since the while loop iterates n times. So it's not the most efficient, but I think from the readability standpoint, it's easy to understand.
This question did get me thinking about arrays and array pointers. There are better, more efficient solutions out there, but for the time being, this solution was the easiest one for me to understand.
One thing I did not do is keep record of the time it took me to solve this problem. I'm hoping to start doing that on the next question I post.
Thanks for your time and thanks for reading my blog!
Cheers,
Rafi